There seems to be a tendency for code writers to vacuum-seal their code. By “vacuum-sealed”, I mean that the code is inconvenient to add, edit, and modify. When you read a section of code it should be as convenient as possible to add a comment, add and remove lines, rearrange the lines, and generally modify the code in any way.
Which of these two bags of coffee is easier to add more coffee to?
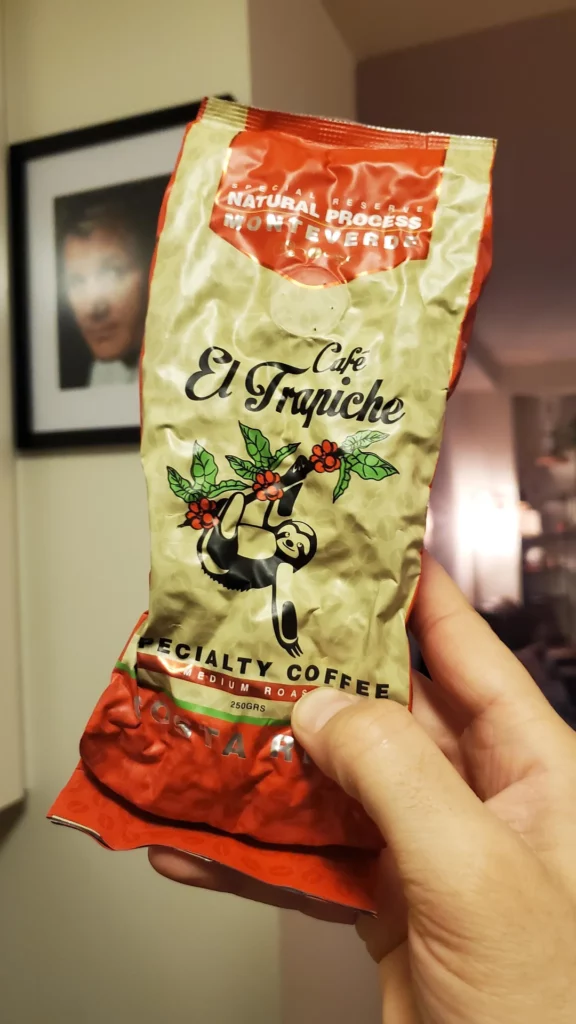
Vacuum-Sealed Coffee bag
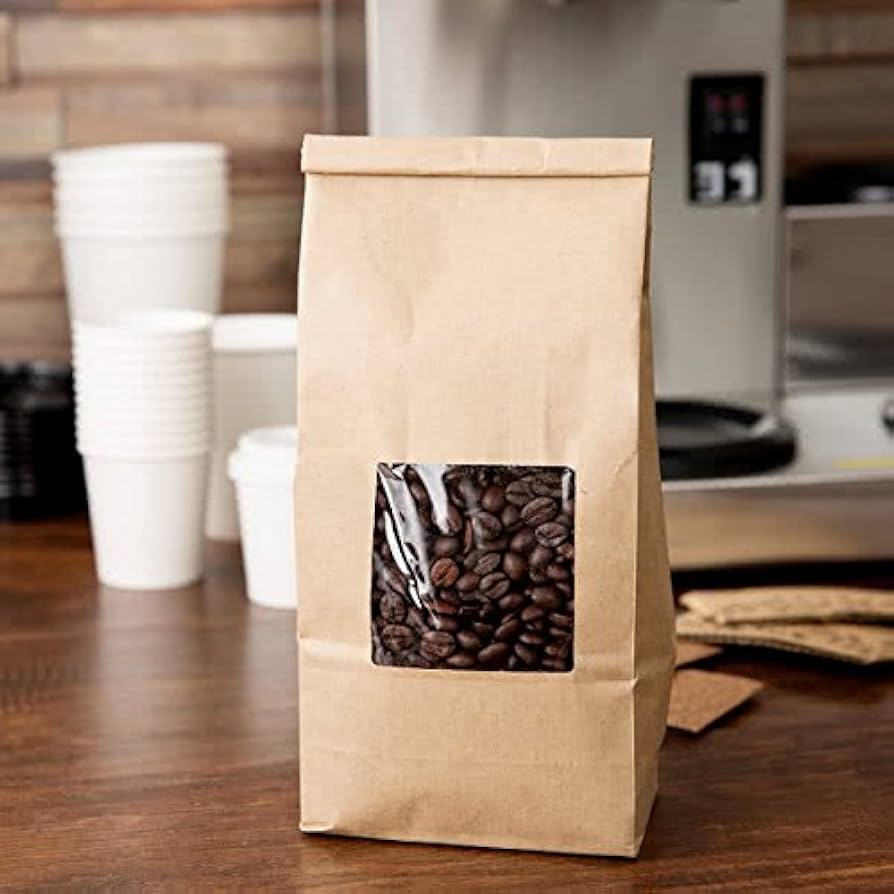
Tin Tied Coffee Bag
It is easier to add coffee to the tin-tied than the vacuum-sealed bag. Don’t vacuum seal the code. Write code that is ‘airy’, uncompressed, and convenient to edit.
Vacuum seals in code are in many forms. Here are a few examples:
Placing all the code in a return statement
... public int someFunction(int input) { return input + someOtherFunction(input) % 2.718; }
This is a fairly short and simple example. This gets really ugly as the function gets into the 10 lines or longer. If you wanted to write a comment on the reason to call ‘someOtherFunction()’ this would be a bit of a hassle; it would be ‘inconvenient’. The refactored code now conveniently allows a comment.
... public int someFunction(int input) { // call someOtherFunction() to spacle over the lower north regiem error. int result = input + someOtherFunction(input); result = result % 2.718; return result; }
This is much more convenient to add the code comment and edit the code. As a by-product, it is easier to debug and step through.
Anonymous Functions
Anonymous Functions are one of the biggest offenders. I continue to be amazed by functional programmers who seem to go to great extremes to avoid writing a function, but this is another post.
... ... iCollection myCollection = theData.Filter(x -> if (n <= 1) return false; for (int i = 2; i * i <= n; i++) if (n % i == 0) return false; return true);
Source of the anonymous function part: https://rosettacode.org/wiki/Primality_by_trial_division#C#
This is also a simple example. In the wild, the anonymous function code gets very long and programmers seem to enter anonymous functions on one line. The anonymous function vacuum seals this code. It is not convenient to fix a bug, add a comment, or write a test.
Also, A first-time reader of this code can not quickly determine what the anonymous function does. Plus, debugging and stepping through the anonymous function is difficult. The code is also not reusable, how would a programmer use the anonymous function in another filter?
The solution to removing the vacuum seal is to simply remove the anonymous and write a function.
... ... iCollection myCollection = theData.Filter(x -> isPrime(x)); ... /// returns true if the input is prime. public Boolean isPrime(Integer input) { if (n <= 1) return false; for (int i = 2; i * i <= n; i++) { if (n % i == 0) return false; } return true; }
In this version the code now has room to breathe, it is more convenient to edit, modify, reuse, debug, and test.
Conclusion
When writing code, try to get a feeling of when the code is vacuum-sealed and rewrite the code to avoid it. This will make your code easier to edit, modify, debug, reuse, and unit test. It will save you time and make future edits in the code convenient.